Prepare for These 9 Critical Java Interview Questions in 2024
With every step in advancing technology, Java remains at the core of software development for large enterprises. No matter if it is your fresh graduation from the Java Course in Coimbatore or even if you’re an experienced professional looking for a role switch; it’s high time you got ready to clear the Java interview round. This definitive guide would let you learn the nine trending questions of 2024 about Java, their complete explanations, and practice examples.
Understanding Java Memory Management and Garbage Collection
Most challenging questions are from Java on memory management, which focuses on garbage collection processes.
Common Interview Question
Explain the working of garbage collection in Java and the different types of garbage collectors present in the modern JVM?
Detailed Answer:
Java’s garbage collection is an automatic memory managing system which identifies and removes all used objects from a running program because they are not being used anymore. It involves the following:
Mark Phase: identifies all the objects which are still in use (referenced).
Sweep Phase: deallocates the memory of unreferenced objects, and it tries to reclaim.
Compact Phase: moves remaining objects to make some free space continuous.
Some other garbage collectors for modern JVM
Serial GC- single thread which is best used for simple application
Parallel GC-Multiple threads would be more beneficial for collection purposes
G1- Garbage first collector designed specifically for large heaps.
ZGC: Low-latency garbage collector introduced in later Java versions
Best Practice Tip:
When choosing a garbage collector, consider your application’s requirements regarding latency, throughput, and heap size.
2. Java Concurrency and Thread Management
As a leading Java Training Institute professional would emphasize, understanding concurrency is crucial for modern applications.
Common Interview Question:
“What’s the difference between synchronized methods and synchronized blocks? When would you use one over the other?”
Detailed Answer:
Synchronized methods and blocks are mechanisms of achieving thread safety in Java but differ in granularity and performance implications:
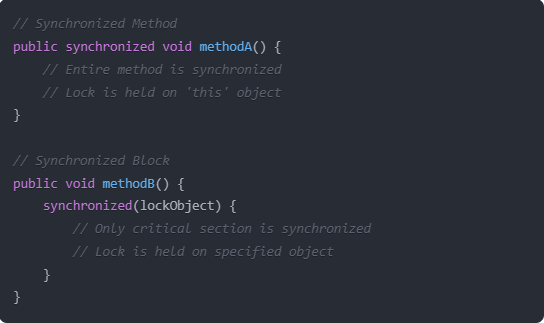
Synchronized blocks have advantages:
Fine-grained control over synchronized code
Less time for a lock
The lock object can be specified
Less chance of deadlocks
3. New Features in Java and Stream API
Common Interview Question:
How do you use Stream API for processing collections, and what are the advantages over traditional iteration methods?
Detailed Answer:
Stream API is a functional way of processing collections in Java 8. Here’s a detailed example:
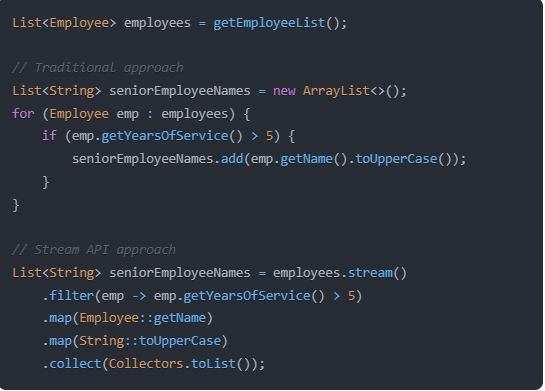
Advantages of Stream API:
- Declarative programming style
- Better readability and maintainability
- Built-in support for parallel processing
- Reduced boilerplate code
4. Design Patterns in Java
Many graduates from a Java Course in Coimbatore find that understanding design patterns is crucial for interviews.
Common Interview Question: Explain the Singleton pattern and its implementation challenges in a multi-threaded environment.
Detailed Answer: The Singleton pattern ensures a class has only one instance while providing global access to it. Here’s a thread-safe implementation:
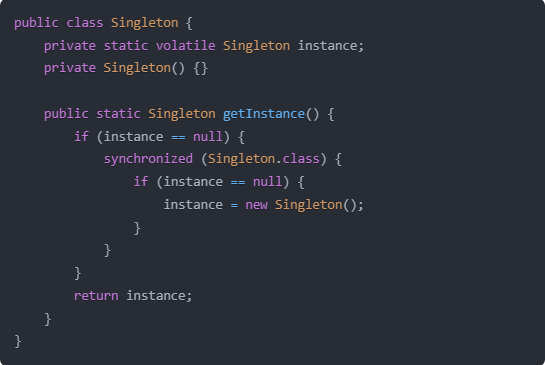
Key considerations:
- Double-checked locking pattern
- Volatile keyword usage
- Private constructor
- Thread safety implications
5. Spring Framework Fundamentals
As any reputable Java Training Institute would teach, Spring Framework knowledge is essential for enterprise Java development.
Common Interview Question: “Explain dependency injection in Spring and its different types.”
Detailed Answer: Dependency Injection (DI) is a core concept in Spring that promotes loose coupling between components. There are three main types:
- Constructor Injection
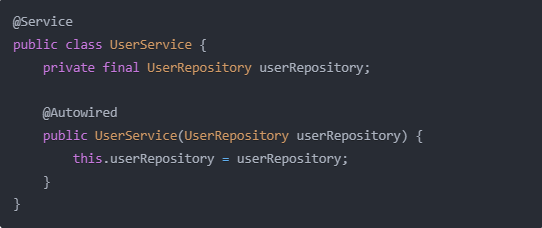
Setter Injection
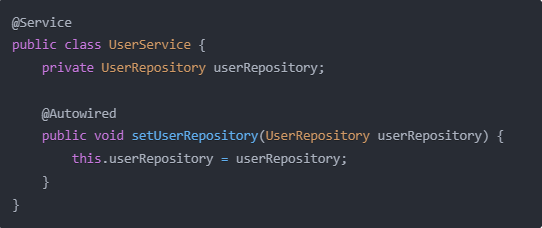
Field Injection
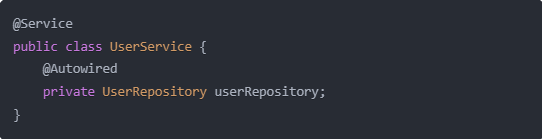
6. Microservices Architecture
Common Interview Question:
How would you implement service discovery and load balancing in a Java microservices architecture?”
Detailed Answer:
Service discovery and load balancing are crucial components of microservices architecture. Here’s a practical implementation using Spring Cloud:
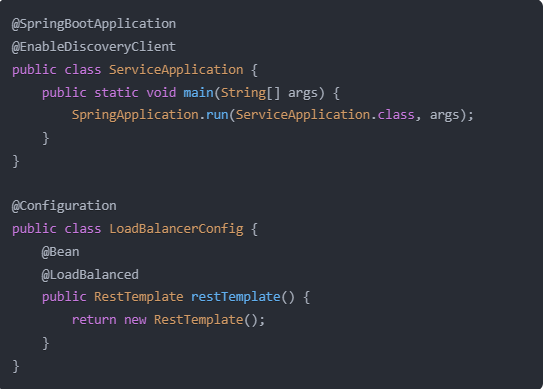
Key components:
- Service registry (Eureka)
- Client-side load balancing (Ribbon)
- Circuit breaker pattern (Hystrix)
- API Gateway (Spring Cloud Gateway)
7. Testing in Java
Common Interview Question:
What are the different types of tests you write in Java, and how do you ensure good test coverage?
Detailed Answer:
A comprehensive testing strategy includes:
// Unit Test Example
@Test
public void testUserServiceCreation() {
User user = new User(“John”, “john@example.com”);
UserService service = new UserService(mockRepository);
when(mockRepository.save(any(User.class))).thenReturn(user);
User result = service.createUser(user);
assertNotNull(result);
assertEquals(“John”, result.getName());
}
// Integration Test Example
@SpringBootTest
public class UserControllerIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testCreateUser() {
UserDTO user = new UserDTO(“John”, “john@example.com”);
ResponseEntity<User> response = restTemplate.postForEntity(“/api/users”, user, User.class);
assertEquals(HttpStatus.CREATED, response.getStatusCode());
}
}
8. Java Performance Optimization
Common Interview Question:
What strategies would you use to optimize a Java application’s performance?”
Detailed Answer:
Performance optimization involves multiple aspects:
- Code Level Optimization
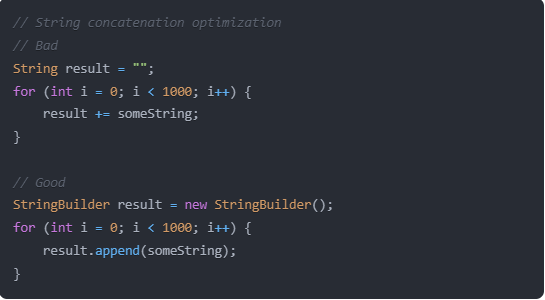
- Memory Management
- Use appropriate collection types
- Implement proper object pooling
- Configure heap size appropriately
- Database Optimization
- Index optimization
- Connection pooling
- Query optimization
9. Security Best Practices
Common Interview Question:
How do you implement secure password storage in Java applications?”
Detailed Answer:
Secure password storage requires multiple security measures:
public class PasswordService {
private static final int ITERATIONS = 65536;
private static final int KEY_LENGTH = 512;
public String hashPassword(String password) throws NoSuchAlgorithmException {
byte[] salt = generateSalt();
PBEKeySpec spec = new PBEKeySpec(
password.toCharArray(),
salt,
ITERATIONS,
KEY_LENGTH
);
SecretKeyFactory skf = SecretKeyFactory.getInstance(“PBKDF2WithHmacSHA512”);
byte[] hash = skf.generateSecret(spec).getEncoded();
return Base64.getEncoder().encodeToString(hash);
}
}
10. Advanced Java Collections Framework
Common Interview Question:
Compare and contrast ConcurrentHashMap with synchronized HashMap. When would you use one over the other?”
Detailed Answer:
Understanding the differences between thread-safe collection implementations is crucial for developing high-performance applications:
// Synchronized HashMap
Map<String, Integer> syncMap = Collections.synchronizedMap(new HashMap<>());
// ConcurrentHashMap
ConcurrentHashMap<String, Integer> concurrentMap = new ConcurrentHashMap<>();
// Usage Example
public class ConcurrentMapExample {
private final ConcurrentHashMap<String, Integer> userScores = new ConcurrentHashMap<>();
public void updateScore(String user, int newScore) {
userScores.compute(user, (key, oldScore) ->
oldScore == null ? newScore : Math.max(oldScore, newScore));
}
public Integer getScore(String user) {
return userScores.getOrDefault(user, 0);
}
}
Key differences:
- ConcurrentHashMap offers better performance for multiple threads
- Segment-level locking instead of object-level
- Atomic operations support
- Null keys and values are not allowed
11. Java Reactive Programming
Common Interview Question: “Explain the benefits of reactive programming in Java and provide an example using Project Reactor.”
Detailed Answer:
Reactive programming is becoming increasingly important for building responsive and resilient applications:
@Service
public class ReactiveUserService {
private final ReactiveUserRepository repository;
public Flux<User> processUsers(Flux<String> userIds) {
return userIds
.flatMap(repository::findById)
.filter(user -> user.getStatus().isActive())
.map(this::enrichUserData)
.doOnNext(this::auditLog)
.onErrorContinue((error, item) ->
log.error(“Error processing user: {}”, item));
}
private User enrichUserData(User user) {
// Enrich user data with additional information
return user.toBuilder()
.withLastAccessTime(LocalDateTime.now())
.build();
}
}
Benefits of reactive programming:
- Non-blocking operations
- Better resource utilization
- Built-in error handling
- Backpressure support
12. Cloud-Native Java Development
Common Interview Question: “How would you design a cloud-native Java application? What considerations are important?”
Detailed Answer: Cloud-native development requires a different approach to application design:
@Configuration
@EnableConfigurationProperties
public class CloudConfig {
@Value(“${cloud.service.endpoint}”)
private String serviceEndpoint;
@Bean
public CloudService cloudService() {
return CloudService.builder()
.endpoint(serviceEndpoint)
.retryConfig(RetryConfig.custom()
.maxAttempts(3)
.build())
.circuitBreaker(CircuitBreaker.custom()
.failureThreshold(5)
.waitDuration(Duration.ofSeconds(30))
.build())
.build();
}
}
Key considerations:
- Configuration Management
- Externalized configuration
- Environment-specific settings
- Secret management
- Resilience Patterns
- Circuit breakers
- Retry mechanisms
- Fallback strategies
- Monitoring and Observability
- Metrics collection
- Distributed tracing
- Log aggregation
13. Modern Build Tools and CI/CD
Common Interview Question: “Compare Gradle with Maven and explain how you would set up a CI/CD pipeline for a Java application.”
Detailed Answer: Modern Java development requires understanding of build tools and automation:
// Gradle build script example
plugins {
id ‘java’
id ‘org.springframework.boot’ version ‘2.7.0’
id ‘io.spring.dependency-management’ version ‘1.0.11.RELEASE’
}
dependencies {
implementation ‘org.springframework.boot:spring-boot-starter-web’
testImplementation ‘org.springframework.boot:spring-boot-starter-test’
implementation platform(‘software.amazon.awssdk:bom:2.17.0’)
implementation ‘software.amazon.awssdk:s3’
}
test {
useJUnitPlatform()
testLogging {
events “passed”, “skipped”, “failed”
}
}
CI/CD Pipeline considerations:
- Automated testing
- Code quality checks
- Security scanning
- Deployment automation
Conclusion
Mastering these nine critical Java interview questions will significantly improve your chances of success in technical interviews. Whether you’re completing a Java Course in Coimbatore or looking to advance your career, understanding these concepts is essential. For comprehensive Java Training that covers all these topics and more, consider xplore IT Corp, where industry experts provide hands-on experience with real-world applications.
Remember that technical interviews are not just about memorizing answers but understanding the underlying concepts and their practical applications. Keep practicing, stay updated with the latest Java developments, and approach each interview as an opportunity to demonstrate your expertise.